Logger
¶
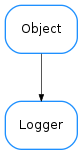
-
class
Logger
(name='', parent=None, format=None)[source]¶ Bases:
taurus.core.util.object.Object
The taurus logger class. All taurus pertinent classes should inherit directly or indirectly from this class if they need taurus logging facilities.
-
Critical
= 50¶ Critical message level (constant)
-
Debug
= 10¶ Debug message level (constant)
-
DftLogFormat
= <logging.Formatter object>¶ Default log format (constant)
-
DftLogLevel
= 20¶ Default log level (constant)
-
DftLogMessageFormat
= '%(threadName)-14s %(levelname)-8s %(asctime)s %(name)s: %(message)s'¶ Default log message format (constant)
-
Error
= 40¶ Error message level (constant)
-
Fatal
= 50¶ Fatal message level (constant)
-
Info
= 20¶ Info message level (constant)
-
Trace
= 5¶ Trace message level (constant)
-
Warning
= 30¶ Warning message level (constant)
-
addLogHandler
(handler)[source]¶ Registers a new handler in this object’s logger
Parameters: handler ( Handler
) – the new handler to be added
-
classmethod
addRootLogHandler
(h)[source]¶ Adds a new handler to the root logger
Parameters: h ( Handler
) – the new log handler
-
changeLogName
(name)[source]¶ Change the log name for this object.
Parameters: name ( str
) – the new log name
-
copyLogHandlers
(other)[source]¶ Copies the log handlers of other object to this object
Parameters: other ( object
) – object which contains ‘log_handlers’
-
critical
(msg, *args, **kw)[source]¶ Record a critical message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.critical()
.Parameters: - msg (
str
) – the message to be recorded - args – list of arguments
- kw – list of keyword arguments
- msg (
-
debug
(msg, *args, **kw)[source]¶ Record a debug message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.debug()
.Parameters: - msg (
str
) – the message to be recorded - args – list of arguments
- kw – list of keyword arguments
- msg (
-
deprecated
(msg=None, dep=None, alt=None, rel=None, dbg_msg=None, _callerinfo=None, **kw)[source]¶ Record a deprecated warning message in this object’s logger. If message is not passed, a estandard deprecation message is constructued using dep, alt, rel arguments. Also, an extra debug message can be recorded, followed by traceback info.
Parameters: - msg (
str
) – the message to be recorded (if None passed, it will be constructed using dep (and, optionally, alt and rel) - dep (
str
) – name of deprecated feature (in case msg is None) - alt (
str
) – name of alternative feature (in case msg is None) - rel (
str
) – name of release from which the feature was deprecated (in case msg is None) - dbg_msg (
str
) – msg for debug (or None to log only the warning) - _callerinfo – for internal use only. Do not use this argument.
- kw – any additional keyword arguments, are passed to
logging.Logger.warning()
- msg (
-
classmethod
disableLogOutput
()[source]¶ Disables the
logging.StreamHandler
which dumps log records, by default, to the stderr.
-
classmethod
enableLogOutput
()[source]¶ Enables the
logging.StreamHandler
which dumps log records, by default, to the stderr.
-
error
(msg, *args, **kw)[source]¶ Record an error message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.error()
.Parameters: - msg (
str
) – the message to be recorded - args – list of arguments
- kw – list of keyword arguments
- msg (
-
exception
(msg, *args)[source]¶ Log a message with severity ‘ERROR’ on the root logger, with exception information.. Accepted args are the same as
logging.Logger.exception()
.Parameters: - msg (
str
) – the message to be recorded - args – list of arguments
- msg (
-
fatal
(msg, *args, **kw)[source]¶ Record a fatal message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.fatal()
.Parameters: - msg (
str
) – the message to be recorded - args – list of arguments
- kw – list of keyword arguments
- msg (
-
getChildren
()[source]¶ Returns the log children for this object
Return type: Logger
Returns: the list of log children
-
classmethod
getLogFormat
()[source]¶ Retuns the current log message format (the root log format)
Return type: str
Returns: the log message format
-
getLogFullName
()[source]¶ Gets the full log name for this object
Return type: str
Returns: the full log name
-
classmethod
getLogLevel
()[source]¶ Retuns the current log level (the root log level)
Return type: int
Returns: a number representing the log level
-
getLogObj
()[source]¶ Returns the log object for this object
Return type: Logger
Returns: the log object
-
getParent
()[source]¶ Returns the log parent for this object or None if no parent exists
Return type: Logger
orNone
Returns: the log parent for this object
-
info
(msg, *args, **kw)[source]¶ Record an info message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.info()
.Parameters: - msg (
str
) – the message to be recorded - args – list of arguments
- kw – list of keyword arguments
- msg (
-
classmethod
initRoot
()[source]¶ Class method to initialize the root logger. Do NOT call this method directly in your code
-
log
(level, msg, *args, **kw)[source]¶ Record a log message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.log()
.Parameters:
-
log_format
= <logging.Formatter object>¶ Default log message format
-
log_level
= 20¶ Current global log level
-
classmethod
removeRootLogHandler
(h)[source]¶ Removes the given handler from the root logger
Parameters: h ( Handler
) – the handler to be removed
-
root_init_lock
= <thread.lock object>¶ Internal usage
-
root_inited
= True¶ Internal usage
-
classmethod
setLogFormat
(format)[source]¶ sets the new log message format
Parameters: level ( str
) – the new log message format
-
classmethod
setLogLevel
(level)[source]¶ sets the new log level (the root log level)
Parameters: level ( int
) – the new log level
-
stack
(target=5)[source]¶ Log the usual stack information, followed by a listing of all the local variables in each frame.
Parameters: target ( int
) – the log level assigned to the recordReturn type: str
Returns: The stack string representation
-
stream_handler
= <logging.StreamHandler object>¶ the main stream handler
-
trace
(msg, *args, **kw)[source]¶ Record a trace message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.log()
.Parameters: - msg (
str
) – the message to be recorded - args – list of arguments
- kw – list of keyword arguments
- msg (
-
traceback
(level=5, extended=True)[source]¶ Log the usual traceback information, followed by a listing of all the local variables in each frame.
Parameters: Return type: Returns: The traceback string representation
-
warning
(msg, *args, **kw)[source]¶ Record a warning message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.warning()
.Parameters: - msg (
str
) – the message to be recorded - args – list of arguments
- kw – list of keyword arguments
- msg (
-